While building out widgets library for OnVoard PopBoxes, I took great inspiration from many existing implementations by companies like Intercom and Zendesk. Not much has been written on building third-party widgets. As such, this post will illustrate some of the key learnings and considerations to evaluate before you serve third-party widgets.
Smart Widgets
As I was looking through implementation examples for widgets, what surprises me was that most widgets that you see on plugins marketplace are what I call Dumb Widgets.
Dumb Widgets are essentially static components that can't be interacted or modified in a programmatic manner. They have no API interface that you can interact with so you are pretty much stuck with the default setup that is provided.
So, why is it so important to provide an API interface to modify config?
Take a look at how we interact with Intercom's chat widget via API below.
On OnVoard's home page, we have 2 widgets that will be shown to visitors on fixed positions.
- GDPR notification widget
- Intercom's chat widget
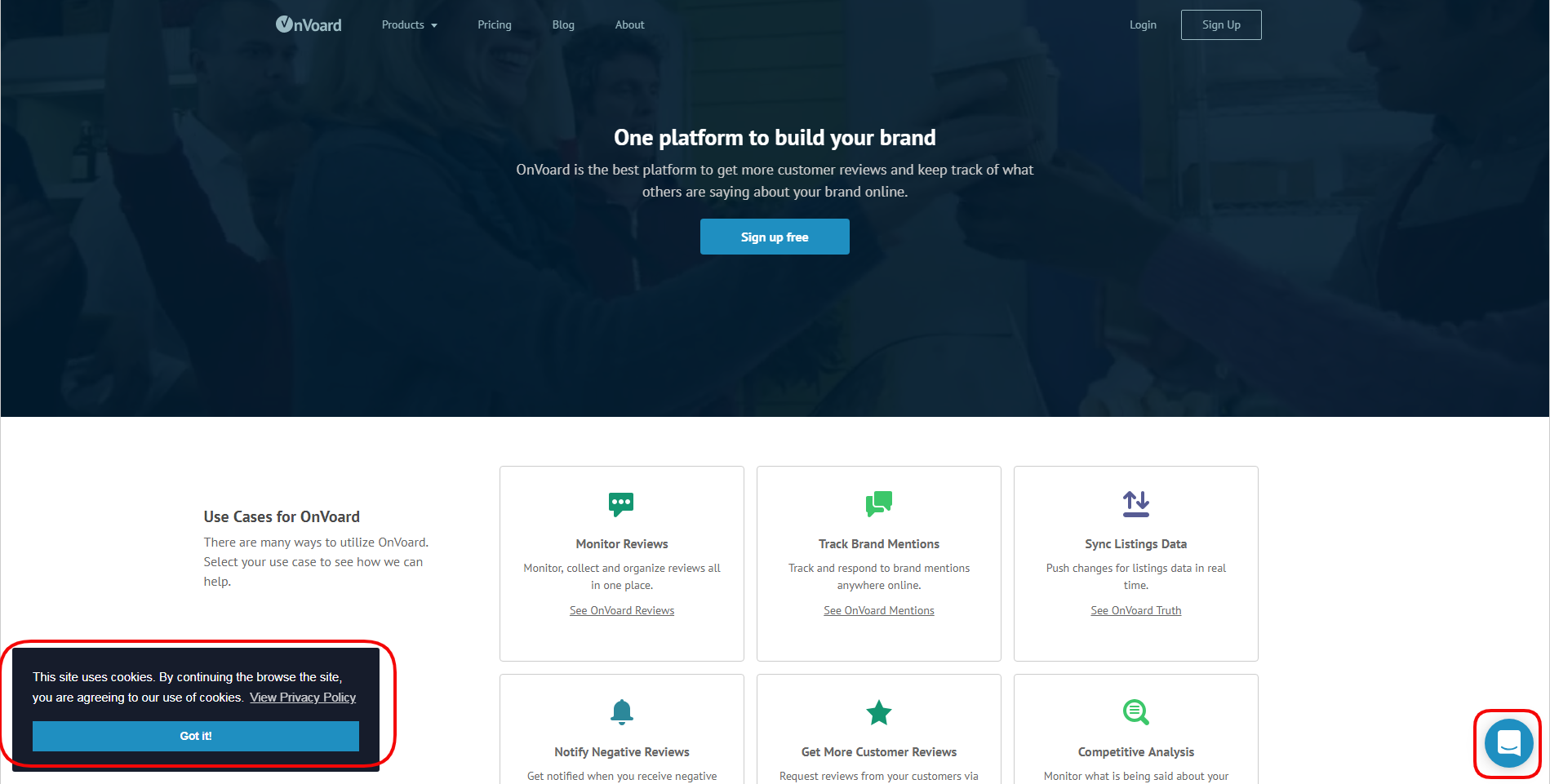
Looks pretty straightforward on desktop right?
Now take a look at how the UX works on a mobile device which has limited screen estate.
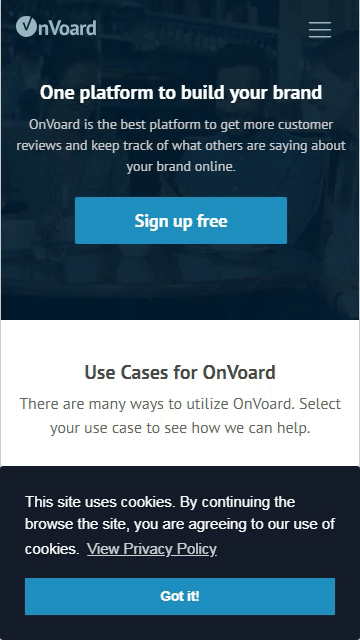
On initial load, we will call Intercom('hide') to hide Intercom's chat widget. But after GDPR's notification has been confirmed, we will call Intercom('show') to show the previously hidden chat widget.
Well, everything looks good when are working with a smart widget similar to the one Intercom provides. But what if Intercom doesn't provide any API interface?
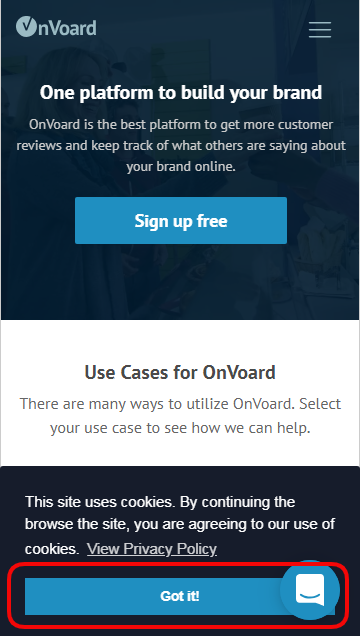
I'm pretty sure everyone has encountered pages with this sort of overlaying issues on their mobile device. And no, z-index isn't the right way to fix this sort of issues because component A may not always fully overlay over component B.
Make it easy to work with your widget. At the very least, you should provide API interface to show or hide your widget.
Consider using loader
One question you need to ask yourself before building out widgets is how many types of widgets do you intend to serve in the future. If you are going to serve multiple widgets, you may want to consider using a loader.
How it works is that instead of requiring your user to place snippets for each widget types, they can add a loader snippet and the loader will determine which widget to load at runtime. This setup works great when you need to serve many different types of widgets.
Below is a sample of widget snippet used in OnVoard.
<script>
window.onvoardConfig = {
popbox: {
id: "YOUR_POPBOX_ID",
on: true
}
};
</script>
<script>
(function() {
window.OnVoard = window.OnVoard || function() {
(window.OnVoard.q = window.OnVoard.q || []).push(arguments);
};
var script = document.createElement("script");
var parent = document.getElementsByTagName("script")[0].parentNode;
script.async = 1;
script.src = "https://widgets.onvoard.io/assets/acct_LKHJuakjh989HKLH.js";
parent.appendChild(script);
})();
</script>
The user can simply add 1 single code snippet to their site and specify widget to display under onvoardConfig variable.
Ideally, structure your code so that users only need to add snippet once.
Command Pattern
One of the commonly used pattern to interact with third-party widgets is the command pattern. Both Intercom and Zendesk use this design for their JavaScript API. Essentially, command pattern allows you to interact with API via a single interface even when you are dealing with multiple sub-resources (widgets).
# show web widget
zE('webWidget', 'show');
# send chat message
zE('webWidget', 'chat:send', 'I\'d like the Jambalaya, please');
# set suggestions for help center
zE('webWidget', 'helpCenter:setSuggestions', { search: 'credit card' });
Examples with Zendesk's API
OnVoard(<namespace>, <method>, <arguments**>)
How OnVoard's schema for command pattern looks like this
OnVoard('popbox', 'reload', {on: false});
OnVoard('reviewsWidget', 'shutdown');
Various examples on how you can make API calls with OnVoard's widget
Pretty neat right? For now, we only provide popbox for now but there are certainly plans to add more widgets in the future.
Versioning
This is where I think most people miss out. If you have no intention to make any changes to your widget then you don't need to use versioning for your widgets. However, most of us will need to make modifications or design changes to widgets. If you are not making changes to a different version, you will eventually break your user's site. Unfortunately, there are very few vendors (Yotpo is one of them) who provide any sort of versioning for their widgets.
For us, what we did was to provide options for the user to nail down on a specific version. Alternatively, the user can opt to stick to the latest version.
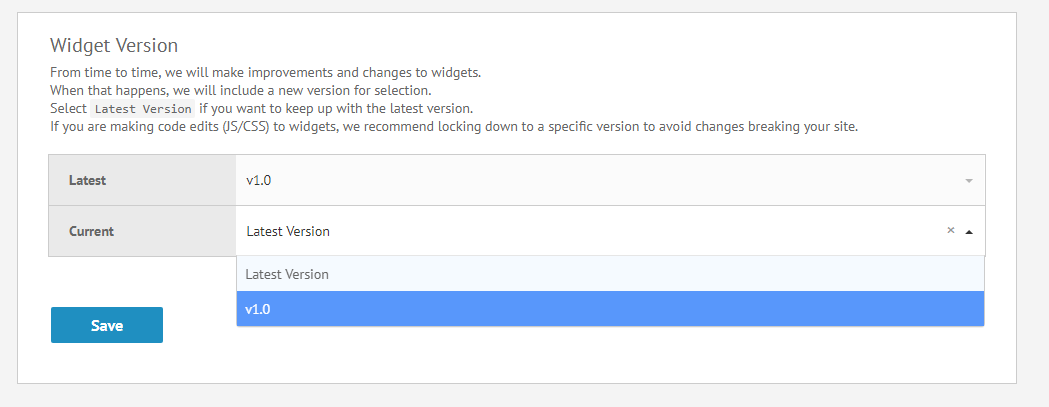
Always provide versioning for your widgets if you can.